Note
Go to the end to download the full example code.
Get specific electrodes and use aliases¶
We need to import some functions
from pprint import pprint
from eeg_positions import (
get_alias_mapping,
get_available_elec_names,
get_elec_coords,
plot_coords,
)
Let’s assume we need only a small subset of electrodes for our work.
We can print all available electrode names in eeg_positions
.
elec_names = get_available_elec_names()
pprint(elec_names[::-1])
['M2',
'M1',
'A2',
'A1',
'NAS',
'RPA',
'LPA',
'POO8h',
'POO6',
'POO6h',
'POO4',
'POO4h',
'POO2',
'POO2h',
'POO1h',
'POO1',
'POO3h',
'POO3',
'POO5h',
'POO5',
'POO7h',
'PO8h',
'PO6',
'PO6h',
'PO4',
'PO4h',
'PO2',
'PO2h',
'PO1h',
'PO1',
'PO3h',
'PO3',
'PO5h',
'PO5',
'PO7h',
'PPO8h',
'PPO6',
'PPO6h',
'PPO4',
'PPO4h',
'PPO2',
'PPO2h',
'PPO1h',
'PPO1',
'PPO3h',
'PPO3',
'PPO5h',
'PPO5',
'PPO7h',
'P8h',
'P6',
'P6h',
'P4',
'P4h',
'P2',
'P2h',
'P1h',
'P1',
'P3h',
'P3',
'P5h',
'P5',
'P7h',
'TPP8h',
'CPP6',
'CPP6h',
'CPP4',
'CPP4h',
'CPP2',
'CPP2h',
'CPP1h',
'CPP1',
'CPP3h',
'CPP3',
'CPP5h',
'CPP5',
'TPP7h',
'TP8h',
'CP6',
'CP6h',
'CP4',
'CP4h',
'CP2',
'CP2h',
'CP1h',
'CP1',
'CP3h',
'CP3',
'CP5h',
'CP5',
'TP7h',
'TTP8h',
'CCP6',
'CCP6h',
'CCP4',
'CCP4h',
'CCP2',
'CCP2h',
'CCP1h',
'CCP1',
'CCP3h',
'CCP3',
'CCP5h',
'CCP5',
'TTP7h',
'FTT8h',
'FCC6',
'FCC6h',
'FCC4',
'FCC4h',
'FCC2',
'FCC2h',
'FCC1h',
'FCC1',
'FCC3h',
'FCC3',
'FCC5h',
'FCC5',
'FTT7h',
'FT8h',
'FC6',
'FC6h',
'FC4',
'FC4h',
'FC2',
'FC2h',
'FC1h',
'FC1',
'FC3h',
'FC3',
'FC5h',
'FC5',
'FT7h',
'FFT8h',
'FFC6',
'FFC6h',
'FFC4',
'FFC4h',
'FFC2',
'FFC2h',
'FFC1h',
'FFC1',
'FFC3h',
'FFC3',
'FFC5h',
'FFC5',
'FFT7h',
'F8h',
'F6',
'F6h',
'F4',
'F4h',
'F2',
'F2h',
'F1h',
'F1',
'F3h',
'F3',
'F5h',
'F5',
'F7h',
'AFF8h',
'AFF6',
'AFF6h',
'AFF4',
'AFF4h',
'AFF2',
'AFF2h',
'AFF1h',
'AFF1',
'AFF3h',
'AFF3',
'AFF5h',
'AFF5',
'AFF7h',
'AF8h',
'AF6',
'AF6h',
'AF4',
'AF4h',
'AF2',
'AF2h',
'AF1h',
'AF1',
'AF3h',
'AF3',
'AF5h',
'AF5',
'AF7h',
'AFp8h',
'AFp6',
'AFp6h',
'AFp4',
'AFp4h',
'AFp2',
'AFp2h',
'AFp1h',
'AFp1',
'AFp3h',
'AFp3',
'AFp5h',
'AFp5',
'AFp7h',
'O1h',
'O1',
'POO7',
'PO7',
'PPO7',
'P7',
'TPP7',
'TP7',
'TTP7',
'FTT7',
'FT7',
'FFT7',
'F7',
'AFF7',
'AF7',
'AFp7',
'Fp1',
'Fp1h',
'O2h',
'O2',
'POO8',
'PO8',
'PPO8',
'P8',
'TPP8',
'TP8',
'TTP8',
'FTT8',
'FT8',
'FFT8',
'F8',
'AFF8',
'AF8',
'AFp8',
'Fp2',
'Fp2h',
'OI1h',
'OI1',
'POO9h',
'PO9h',
'PPO9h',
'P9h',
'TPP9h',
'TP9h',
'TTP9h',
'FTT9h',
'FT9h',
'FFT9h',
'F9h',
'AFF9h',
'AF9h',
'AFp9h',
'NFp1',
'NFp1h',
'OI2h',
'OI2',
'POO10h',
'PO10h',
'PPO10h',
'P10h',
'TPP10h',
'TP10h',
'TTP10h',
'FTT10h',
'FT10h',
'FFT10h',
'F10h',
'AFF10h',
'AF10h',
'AFp10h',
'NFp2',
'NFp2h',
'T10h',
'T8',
'T8h',
'C6',
'C6h',
'C4',
'C4h',
'C2',
'C2h',
'C1h',
'C1',
'C3h',
'C3',
'C5h',
'C5',
'T7h',
'T7',
'T9h',
'I1h',
'I1',
'POO9',
'PO9',
'PPO9',
'P9',
'TPP9',
'TP9',
'TTP9',
'T9',
'FTT9',
'FT9',
'FFT9',
'F9',
'AFF9',
'AF9',
'AFp9',
'N1',
'N1h',
'I2h',
'I2',
'POO10',
'PO10',
'PPO10',
'P10',
'TPP10',
'TP10',
'TTP10',
'T10',
'FTT10',
'FT10',
'FFT10',
'F10',
'AFF10',
'AF10',
'AFp10',
'N2',
'N2h',
'Iz',
'OIz',
'Oz',
'POOz',
'POz',
'PPOz',
'Pz',
'CPPz',
'CPz',
'CCPz',
'Cz',
'FCCz',
'FCz',
'FFCz',
'Fz',
'AFFz',
'AFz',
'AFpz',
'Fpz',
'NFpz',
'Nz']
A keen eye may have identified some “odd” electrodes, that are not really part
of the 10-20, 10-10, or 10-05 systems: 'A1', 'A2', 'M1', 'M2'
These are supplied with eeg_positions
for convenience as so-called “alias”
positions. See below:
alias_mapping = get_alias_mapping()
for key, val in alias_mapping.items():
print(f"{key}: {val}")
A1: LPA+(-0.1, -0.01, -0.01)
A2: RPA+(0.1, -0.01, -0.01)
M1: TP9
M2: TP10
We see that for example A1 is mapped to a position near the left preauricular point (LPA). This position is meant to reflect that the A1 electrode is typically a clip-on electrode applied to the earlobe.
Similarly, the M1 electrode is typically applied to the left mastoid on the head of a study participant. Its position approximately coincides with the TP9 position.
But now back to the topic: We want to get coordinates for a small subset of electrodes!
# Just use `elec_names`
coords = get_elec_coords(
elec_names=["A1", "A2", "FC3", "CP4", "M1", "LPA", "RPA", "T10"],
drop_landmarks=False,
dim="3d",
)
coords.head()
Plot it, and see how close LPA and A1 (and RPA and A2) are in space.
fig, ax = plot_coords(coords, text_kwargs=dict(fontsize=10))
ax.axis("off")
fig
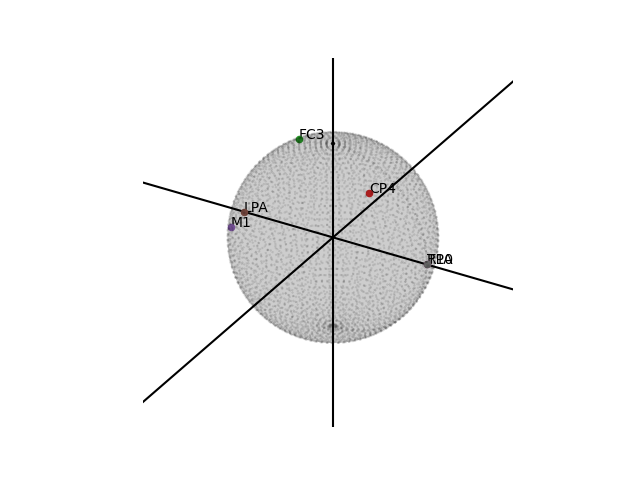
<Figure size 640x480 with 1 Axes>